Taobao Data Source Analysis and Technical Selection: API vs Web Scraping vs Hybrid Crawling
1. Introduction
When building an e-commerce data platform or analysis system, one of the most critical technical challenges is:
How to obtain large-scale, accurate product data from Taobao efficiently and reliably?
While Taobao offers official APIs, they come with restrictions like authorization, rate limits, and access scope. On the other hand, web scraping is more flexible but must navigate anti-bot mechanisms and page structure variability. A hybrid approach, combining both API and scraping, can help balance reliability and data coverage.
In this article, we will:
Analyze and compare three main data acquisition strategies
Provide real Python examples: calling Taobao APIs, scraping Taobao pages, and fallback logic in hybrid architecture
Offer practical technical selection guidance for real-world applications
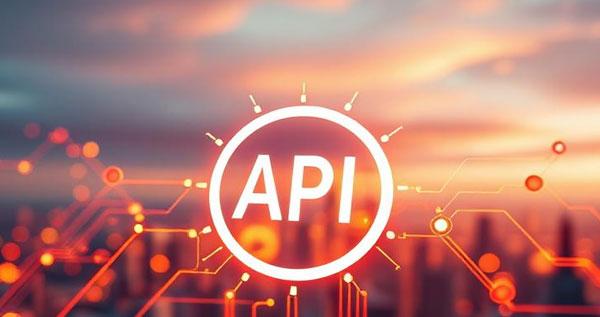
2. Overview of the Three Major Data Acquisition Methods
Method | Advantages | Disadvantages | Best Use Case |
---|---|---|---|
Official API | Legal, structured data, stable, well-documented | Rate limits, requires app approval, incomplete field access | Stable, formal systems |
Web Scraping | Highly flexible, access any public information | Anti-bot risk, fragile to UI changes, messy HTML structure | Large-scale, unstructured data |
Hybrid | Best of both worlds, balanced between coverage & control | Complex implementation, requires maintaining both systems | High-reliability + full coverage |
3. Method 1: Using the Official API
3.1 Core Idea
Use Taobao’s developer platform and call their open APIs with proper authorization and signed requests to get structured product data like title, price, sales, inventory, etc.
3.2 Pros & Cons
Pros:
Stable and structured data
Official support with documentation and error codes
Supports incremental queries and filtering
Cons:
Requires AppKey/AppSecret and approval
Rate limited (QPS, daily limits)
Some fields or endpoints are restricted or unavailable
3.3 Python Example
import requests, hashlib, timeAPI_URL = 'https://api.taobao.com/router/rest'
API_KEY = 'YOUR_APP_KEY'
API_SECRET = 'YOUR_APP_SECRET'
def generate_signature(params):
sorted_keys = sorted(params.keys())
base = API_SECRET + ''.join(f"{k}{params[k]}" for k in sorted_keys) + API_SECRET
return hashlib.md5(base.encode('utf-8')).hexdigest().upper()
def fetch_item_detail(num_iid):
params = {
'method': 'taobao.item.get',
'app_key': API_KEY,
'timestamp': time.strftime('%Y-%m-%d %H:%M:%S'),
'format': 'json',
'v': '2.0',
'num_iid': num_iid,
'fields': 'title,price,volume,detail_url'
}
params['sign'] = generate_signature(params)
resp = requests.get(API_URL, params=params)
return resp.json()
if __name__ == '__main__':
detail = fetch_item_detail(1234567890123)
print(detail)
Explanation:
generate_signature
follows Taobao's rules to sign parametersThe response includes structured product data
You may use caching or rotate multiple API accounts if limits are exceeded
4. Method 2: Web Scraping
4.1 Core Idea
Send HTTP requests directly to Taobao’s search or product detail pages, then parse HTML content using tools like BeautifulSoup or XPath to extract the required information.
4.2 Pros & Cons
Pros:
No need for authorization
Can access any data visible in the frontend
Cons:
Subject to anti-bot defenses (e.g., IP bans, CAPTCHA)
HTML structure changes frequently
Parsing logic can be fragile and maintenance-heavy
4.3 Python Scraping Example
import requestsfrom bs4 import BeautifulSoup
import time
HEADERS = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 ...'
}
def scrape_search(keyword, page=1):
url = f'https://s.taobao.com/search?q={keyword}&s={(page-1)*44}'
resp = requests.get(url, headers=HEADERS, timeout=10)
soup = BeautifulSoup(resp.text, 'lxml')
items = soup.select('.J_MouserOnverReq')
results = []
for it in items:
title = it.select_one('.J_ClickStat').get('title', '').strip()
price = it.select_one('.price').get_text().strip()
link = 'https:' + it.select_one('.pic-link').get('href')
results.append({'title': title, 'price': price, 'link': link})
return results
if __name__ == '__main__':
data = scrape_search('bluetooth earbuds', page=1)
for d in data[:5]:
print(d)
time.sleep(1)
Explanation:
Custom
User-Agent
helps simulate real browsersUse CSS selectors to locate product elements
Throttle requests and consider using proxies for safety
5. Method 3: Hybrid Data Acquisition Strategy
5.1 Core Idea
Use official APIs for structured and stable data retrieval
Fallback to web scraping only when API limits are hit or the required fields are missing
5.2 Pros & Cons
Pros:
Balance between stability (API) and flexibility (scraping)
Ensure core data is covered reliably
Maximizes data completeness
Cons:
Requires managing two systems
Increases development and maintenance complexity
5.3 Python Hybrid Example
def get_item_info(num_iid):try:
# Attempt API first
detail = fetch_item_detail(num_iid)
if 'item_get_response' in detail:
return detail['item_get_response']['item']
except Exception as e:
print('API failed, falling back to scraping:', e)
# Fallback to scraper
return scrape_detail_page(num_iid)
def scrape_detail_page(num_iid):
url = f'https://item.taobao.com/item.htm?id={num_iid}'
resp = requests.get(url, headers=HEADERS, timeout=10)
soup = BeautifulSoup(resp.text, 'lxml')
title = soup.select_one('#J_Title .tb-main-title').get('data-title')
price = soup.select_one('.tb-rmb-num').get_text().strip()
return {'num_iid': num_iid, 'title': title, 'price': price}
Explanation:
get_item_info
prioritizes the API callIf API fails or is rate-limited, it gracefully switches to the scraper
In production, add retry logic, monitoring, and logging
6. Decision-Making Guide for Technical Selection
→ Analyze business goals→ Evaluate API coverage vs required fields
→ Estimate frequency and volume
→ Compare implementation & maintenance cost
→ Choose between API / scraping / hybrid
If stability matters most, go with API first
If data diversity is key, consider scraping or hybrid
For large-scale systems, hybrid gives best flexibility
For quick MVPs or one-off jobs, scraping may be faster
7. Conclusion
This article presented a detailed comparison of three data acquisition approaches for Taobao:
Official API for structure and reliability
Web Scraping for flexibility and full visibility
Hybrid Strategy for robustness and completeness
We explored each method’s pros/cons, showed real Python implementations, and provided a step-by-step decision guide. With this foundation, you’re now equipped to build scalable Taobao data pipelines tailored to your needs.
Articles related to APIs :
Introduction to Taobao API: Basic Concepts and Application Scenarios
Taobao API: Authentication & Request Flow Explained with Code Examples
Using the Taobao API to Retrieve Product Information and Implement Keyword Search
How to Use the Taobao API to Build a Product Price Tracker and Alert System
Using the Taobao API to Build a Category-Based Product Recommendation System
If you need the Taobao API, feel free to contact us : support@luckdata.com